Student achievement
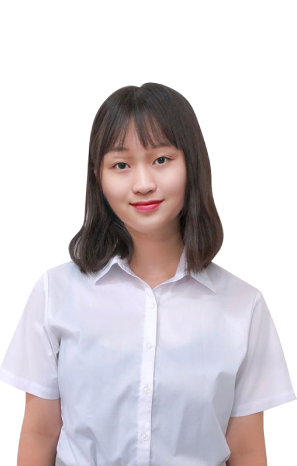
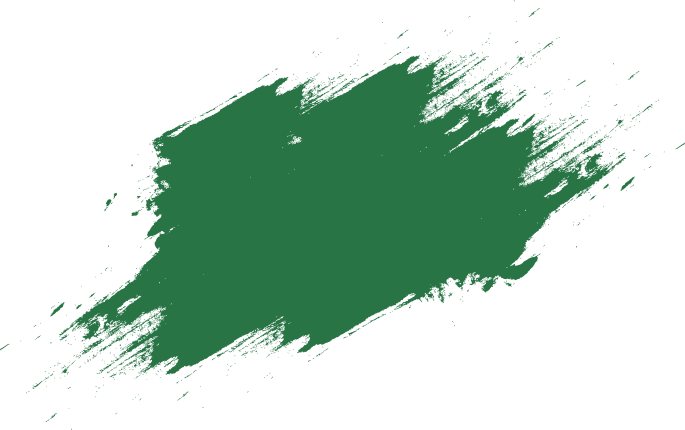
Student's name
Lam Ngoc Thao Lam
Subject
Score:
IB Math AA HL
7/7
IB Physics HL
7/7
IB Chemistry HL
7/7
IB Economics
6/7

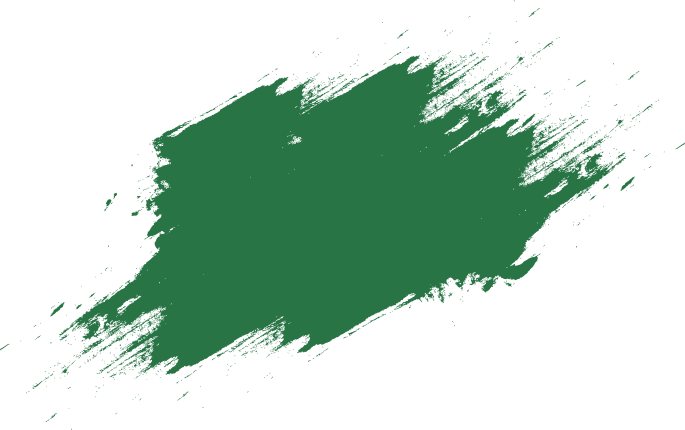
Student's name
Vo Le Minh Son
Subject
Score:
IB Math AI HL
6/7
IB Economics HL
6/7
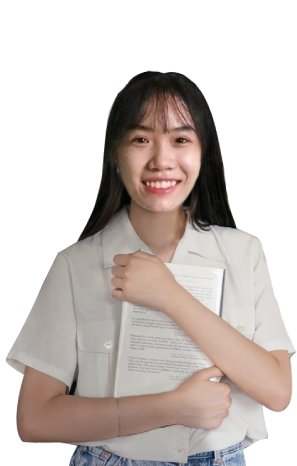
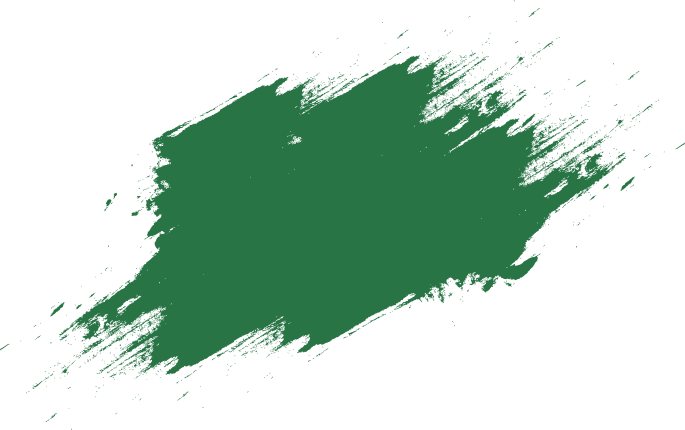
Student's name
Huynh Thien Thanh
Subject
Score:
IB Math AA SL
6/7
IB Chemistry SL
6/7
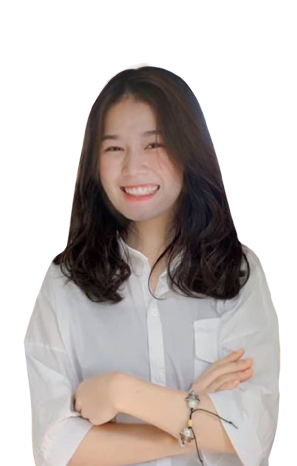
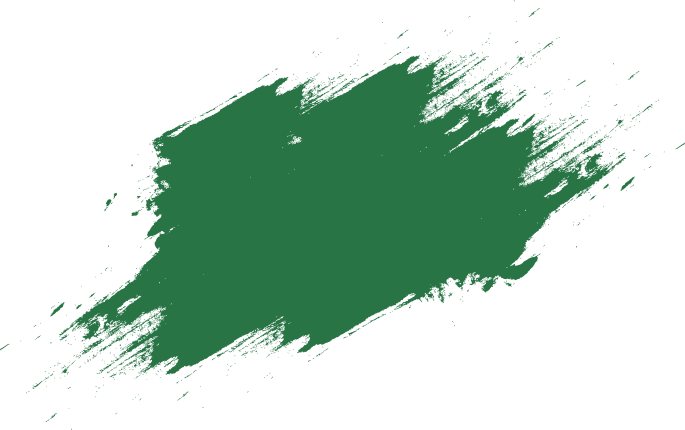
Student's name
Nguyen Ha Dieu Anh
Subject
Score:
A Level Physics
B
A level Economics
A
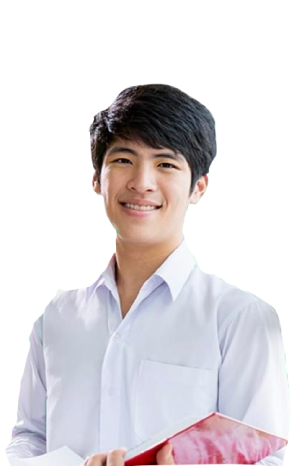
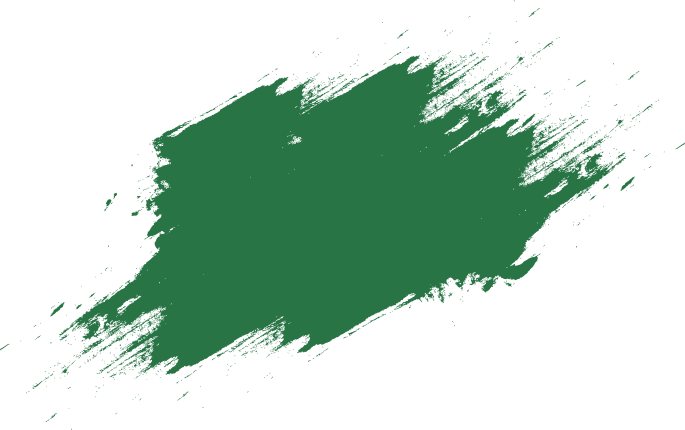
Student's name
Luu Anh Vu
Subject
Score:
A Level Math
A